Here is a workaround for ASP.NET C# if you want an event that triggers on pressing the "Enter" key on your keyboard while still inside a textbox.
First the javascript snippet:
if (event.which || event.keyCode) {
if ((event.which == 13) || (event.keyCode == 13)) {
document.getElementById('btnClick').click();
return false;
}
}
else {
return true;
};
It just captures the "Enter" key press (which is 13) and then clicks the corresponding button to trigger its event. So you just place this script on the textbox's onkeydown attribute which I'll show in just a sec.
protected void Page_Load(object sender, EventArgs e)
{
if (!IsPostBack)
{
txtBox.Attributes.Add("onkeydown", "if(event.which || event.keyCode){if ((event.which == 13) || (event.keyCode == 13)) {document.getElementById('" + btnClick.UniqueID + "').click();return false;}} else {return true}; ");
}
}
Just add the attribute on the Page_Load event and you're done.
Check this link for more keycodes: http://www.cambiaresearch.com/c4/702b8cd1-e5b0-42e6-83ac-25f0306e3e25/Javascript-Char-Codes-Key-Codes.aspx
-k
Thursday, February 25, 2010
ASP.NET C# OnEnter event in TextBox
ASP.NET C# : Working With Cookies
If enabled on client browsers, cookies are a great way to store bits of data for use by websites like remembering the login state of users who doesn't want to retype their username and password everytime they visit the site.
Here are the most common functions you can use to manipulate cookies in ASP.NET (C#):
SETCOOKIE
public void SetCookie(string name, string value, int expiration)
{
HttpCookie cookie;
if (Request.Cookies[name] == null) cookie = new HttpCookie(name);
else cookie = Request.Cookies[name];
cookie.Value = value;
cookie.Expires = DateTime.Now.AddDays(expiration);
Response.Cookies.Add(cookie);
}
SetCookie can be used to add a new cookie or overwrite an exisiting one in your cookie collection. Simply pass the name and value pair and the expiration (in days) for how long you want that cookie to be in use by your website. In the following code, we store the username "abby" in our collection for 1 day.
SetCookie("username", "abby", 1);
Take note though that if you use the same cookie name "username" again to set another value, it will overwrite the previous one.
GETCOOKIE
public string GetCookie(string name)
{
HttpCookie cookie = Request.Cookies[name];
if (cookie == null) return "";
else return cookie.Value;
}
GetCookie can be used to retrieve the value of the cookie by passing the name. In the following code, we get the value of the cookie considering it has not yet expired.
string str = GetCookie("username");
DELETECOOKIE
public void DeleteCookie(string name)
{
HttpCookie cookie;
if (Request.Cookies[name] == null) return;
else cookie = Request.Cookies[name];
cookie.Expires = DateTime.Now.AddDays(-1);
Response.Cookies.Add(cookie);
}
DeleteCookie can be used to delete a particular cookie from the cookie collection by passing the name. Notice the -1 in the AddDays? That will simply delete the value in the physical cookie file. In the following code, we delete the "username" cookie.
DeleteCookie("username");
CLEARCOOKIES
public void ClearCookies()
{
int cookieCount = Request.Cookies.Count;
for (int i = 0; i < cookieCount; i++)
{
HttpCookie cookie = Request.Cookies[i];
cookie.Expires = DateTime.Now.AddDays(-1);
Response.Cookies.Add(cookie);
}
}
ClearCookies can be used to delete all cookies in the cookie collection. We also used the -1 in the AddDays here but used a loop to delete each one in the collection. In the following code, it will delete the physical cookie file of your website.
ClearCookies();
And that's it for cookies.
-k
Here are the most common functions you can use to manipulate cookies in ASP.NET (C#):
SETCOOKIE
public void SetCookie(string name, string value, int expiration)
{
HttpCookie cookie;
if (Request.Cookies[name] == null) cookie = new HttpCookie(name);
else cookie = Request.Cookies[name];
cookie.Value = value;
cookie.Expires = DateTime.Now.AddDays(expiration);
Response.Cookies.Add(cookie);
}
SetCookie can be used to add a new cookie or overwrite an exisiting one in your cookie collection. Simply pass the name and value pair and the expiration (in days) for how long you want that cookie to be in use by your website. In the following code, we store the username "abby" in our collection for 1 day.
SetCookie("username", "abby", 1);
Take note though that if you use the same cookie name "username" again to set another value, it will overwrite the previous one.
GETCOOKIE
public string GetCookie(string name)
{
HttpCookie cookie = Request.Cookies[name];
if (cookie == null) return "";
else return cookie.Value;
}
GetCookie can be used to retrieve the value of the cookie by passing the name. In the following code, we get the value of the cookie considering it has not yet expired.
string str = GetCookie("username");
DELETECOOKIE
public void DeleteCookie(string name)
{
HttpCookie cookie;
if (Request.Cookies[name] == null) return;
else cookie = Request.Cookies[name];
cookie.Expires = DateTime.Now.AddDays(-1);
Response.Cookies.Add(cookie);
}
DeleteCookie can be used to delete a particular cookie from the cookie collection by passing the name. Notice the -1 in the AddDays? That will simply delete the value in the physical cookie file. In the following code, we delete the "username" cookie.
DeleteCookie("username");
CLEARCOOKIES
public void ClearCookies()
{
int cookieCount = Request.Cookies.Count;
for (int i = 0; i < cookieCount; i++)
{
HttpCookie cookie = Request.Cookies[i];
cookie.Expires = DateTime.Now.AddDays(-1);
Response.Cookies.Add(cookie);
}
}
ClearCookies can be used to delete all cookies in the cookie collection. We also used the -1 in the AddDays here but used a loop to delete each one in the collection. In the following code, it will delete the physical cookie file of your website.
ClearCookies();
And that's it for cookies.
-k
Saturday, February 13, 2010
IIS7 Publish Problems with Database
So after testing your website on Visual Studio and made sure everything is working fine, especially database connections, you now published your new site on IIS7 and to your surprise connections don't work as you've set. Try these following steps..
Open IIS by going to Control Panel > Administrative Tools > Internet Information Services (IIS) Manager. On the left menu, click on Application Pools.
Click on the Identity selector under Process Model.
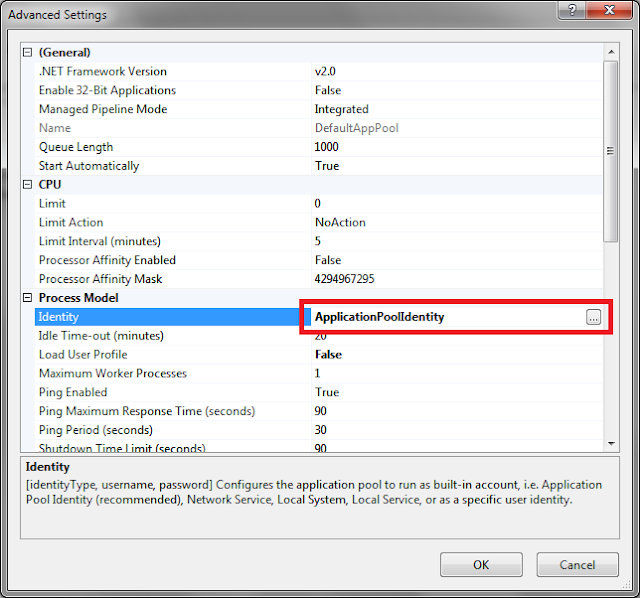
Open IIS by going to Control Panel > Administrative Tools > Internet Information Services (IIS) Manager. On the left menu, click on Application Pools.
Click on DefaultAppPool and then Advanced Settings...
Click on the Identity selector under Process Model.
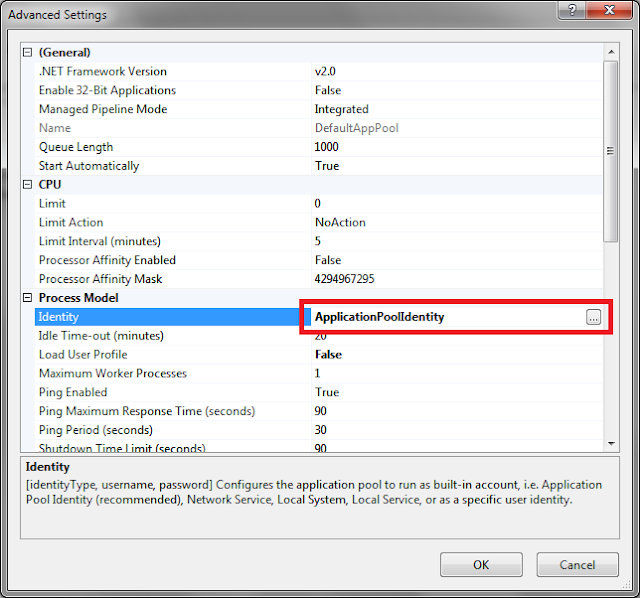
Change it to LocalSystem and click Ok.
Now try running your website again and it should have no more connection problems.
-k
Thursday, February 11, 2010
Get Client MAC Address & IP Address using Javascript
This is somewhat similar to the previous post Get client cpu id with javascript, so the client still needs to enable ActiveX components on his browser. Read more on the said post for details.
Ok if you're done reading, you'll notice we simply change the script to this:
<script type="text/javascript">
var macAddress = "";
var ipAddress = "";
var computerName = "";
var wmi = GetObject("winmgmts:{impersonationLevel=impersonate}");
e = new Enumerator(wmi.ExecQuery("SELECT * FROM Win32_NetworkAdapterConfiguration WHERE IPEnabled = True"));
for(; !e.atEnd(); e.moveNext()) {
var s = e.item();
macAddress = s.MACAddress;
ipAddress = s.IPAddress(0);
computerName = s.DNSHostName;
}
</script>
Instead of Win32_Processor, here we'll access Win32_NetworkAdapterConfiguration to read network related details like the MAC Address, IP Address and the computer name.
Then we can simply use textboxes to display that information or whatever you like.
<input type="text" id="txtMACAdress" />
<input type="text" id="txtIPAdress" />
<input type="text" id="txtComputerName" />
<script type="text/javascript">
document.getElementById("txtMACAdress").value = unescape(macAddress);
document.getElementById("txtIPAdress").value = unescape(ipAddress);
document.getElementById("txtComputerName").value = unescape(computerName);
</script>
And just like before, make sure to place the declarations snippet above this script for it to display the values properly. Now the client can see his/her own MAC and IP Addresses.
Read more about the Win32_NetworkAdapterConfiguration object here http://msdn.microsoft.com/en-us/library/aa394217(VS.85).aspx
-k
Ok if you're done reading, you'll notice we simply change the script to this:
<script type="text/javascript">
var macAddress = "";
var ipAddress = "";
var computerName = "";
var wmi = GetObject("winmgmts:{impersonationLevel=impersonate}");
e = new Enumerator(wmi.ExecQuery("SELECT * FROM Win32_NetworkAdapterConfiguration WHERE IPEnabled = True"));
for(; !e.atEnd(); e.moveNext()) {
var s = e.item();
macAddress = s.MACAddress;
ipAddress = s.IPAddress(0);
computerName = s.DNSHostName;
}
</script>
Instead of Win32_Processor, here we'll access Win32_NetworkAdapterConfiguration to read network related details like the MAC Address, IP Address and the computer name.
Then we can simply use textboxes to display that information or whatever you like.
<input type="text" id="txtMACAdress" />
<input type="text" id="txtIPAdress" />
<input type="text" id="txtComputerName" />
<script type="text/javascript">
document.getElementById("txtMACAdress").value = unescape(macAddress);
document.getElementById("txtIPAdress").value = unescape(ipAddress);
document.getElementById("txtComputerName").value = unescape(computerName);
</script>
And just like before, make sure to place the declarations snippet above this script for it to display the values properly. Now the client can see his/her own MAC and IP Addresses.
Read more about the Win32_NetworkAdapterConfiguration object here http://msdn.microsoft.com/en-us/library/aa394217(VS.85).aspx
-k
Labels:
activex,
computer name,
html,
ip address,
javascript,
mac address
Wednesday, February 10, 2010
ASP.NET AJAX this._form is null error on javascript
If ever you encounter this sneaky error on your ASP.NET AJAX-Enabled websites (you would know it when you see the this._form is null javascript error message or simply if your controls don't cause postbacks anymore) the culprit is the javascript declaration found on the head tag in the html page.
If you have declared it like this:
<script src="scripts/main.js" type="text/javascript" />
Simply change it to this:
<script src="scripts/main.js" type="text/javascript"></script>
And problem is solved.
-k
If you have declared it like this:
<script src="scripts/main.js" type="text/javascript" />
Simply change it to this:
<script src="scripts/main.js" type="text/javascript"></script>
And problem is solved.
-k
Subscribe to:
Posts (Atom)